안녕하세요. 두부입니다.
이번엔 자바로 파일을 복사하는 방법에 대해서 포스팅해보려고합니다.
자바로 파일을 복사하는 4가지 방법을 알려드릴텐데 아래 방식만 알아도 파일을 복사하는데는 큰 어려움이 없을 것이라고 생각합니다.
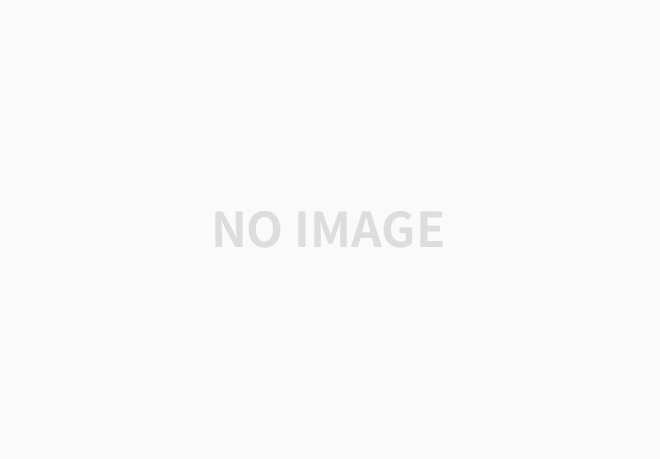
현재 C:/test 경로에 excel_test.cell 이라는 파일이 있습니다. 이 파일을 4가지 방법으로 복사해보겠습니다.
자바로 파일 복사하는 방법
1. FileInputStream / FileOutputStream
import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; public class Main { public static void main(String[] args) throws IOException { String orgFilePath = "C:/test/excel_test.cell"; String copyFilePath = "C:/test/excel_copy.cell"; try { FileInputStream fis = new FileInputStream(orgFilePath); FileOutputStream fos = new FileOutputStream(copyFilePath); byte[] b = new byte[1024]; // 버퍼 생성 int len; // 버퍼에 데이터를 담아서 0번째 offset부터 1024 길이만큼 FileOutputStream에 쓴다. while ((len = fis.read(b, 0, 1024)) > 0) { fos.write(b, 0, len); } fis.close(); fos.close(); } catch (Exception e) { System.out.println(e.getMessage()); } } }
가장 오래된 방법으로 I/O 을 사용해서 버퍼로 읽은 총 byte 수를 복사하는 방법입니다.
2. Files.Copy
import java.io.File; import java.io.IOException; import java.nio.file.Files; public class Main { public static void main(String[] args) throws IOException { String orgFilePath = "C:/test/excel_test.cell"; String copyFilePath = "C:/test/excel_copy.cell"; try { File orgFile = new File(orgFilePath); File copyFile = new File(copyFilePath); Files.copy(orgFile.toPath(), copyFile.toPath()); } catch (Exception e) { System.out.println(e.getMessage()); } } }
java.nio 패키지를 이용한 파일 복사 방법입니다.
Files.copy(Path source, Path target, options);
⑴ Path source: 복사할 파일
⑵ Path target: 복사된 파일
⑶ options
┕ REPLACE_EXISTING: target 파일이 존재하면 덮어쓴다.
┕ COPY_ATTRIBUTES: 파일 attribute를 복사한다.
┕ NOFOLLOW_LINKS: 파일이 sysmbolic link이면 링크 대상이 아닌 symbolic link 자체가 복사된다.
3. FileChannel
import java.io.IOException; import java.io.RandomAccessFile; import java.nio.channels.FileChannel; public class Main { public static void main(String[] args) throws IOException { String orgFilePath = "C:/test/excel_test.cell"; String copyFilePath = "C:/test/excel_copy.cell"; try { RandomAccessFile orgFile = new RandomAccessFile(orgFilePath, "r"); RandomAccessFile copyFile = new RandomAccessFile(copyFilePath, "rw"); FileChannel source = orgFile.getChannel(); FileChannel target = copyFile.getChannel(); // source.transferTo(0, source.size(), target); // target.transferFrom(source, 0, source.size()); } catch (Exception e) { System.out.println(e.getMessage()); } } }
FileChannel 클래스는 파일의 읽기, 쓰기 등을 위한 채널을 제공합니다.
transferTo(), transferFrom() 메소드 두 가지가 존재합니다. 필요에 따라 구분해서 사용하시면 됩니다.
// src 채널로부터 position 위치부터 count 사이즈만큼 복사
source.transferTo(ReadableByteChannel src, long position, long count);
// target 채널로 position 위치부터 count 사이즈만큼 복사
target.transferFrom(long position, long count, WritableByteChannel target);
이 메소드의 경우에는 길이만큼 복사해주는 것이기 때문에 target 파일이 이미 존재하고 아래와 같이 데이터가 들어있다면 예외가 발생한다.
- source 파일: "1234"
- target 파일: "5678910"
target 파일: "1234910" 이 된다.
4. FileUtils (Apache Commons IO)
<dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.11.0</version> </dependency>
import org.apache.commons.io.FileUtils; import java.io.IOException; public class Main { public static void main(String[] args) throws IOException { String orgFilePath = "C:/test/excel_test.cell"; String copyFilePath = "C:/test/excel_copy.cell"; try { File orgFile = new File(orgFilePath); File copyFile = new File(copyFilePath); FileUtils.copyFile(orgFile, copyFile); } catch (Exception e) { System.out.println(e.getMessage()); } } }
Apache Commons IO 라이브러리를 사용하기 때문에 pom.xml에 dependency를 추가해줘야 사용이 가능합니다.
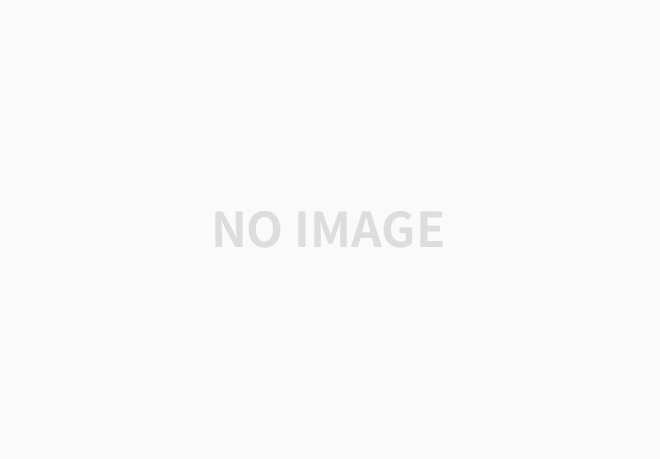
'개발 & 데이터베이스 > JAVA' 카테고리의 다른 글
자바 String, StringBuffer, StringBuilder 차이 (0) | 2022.11.12 |
---|---|
자바 정적 (Static) 메서드 정의 및 생성, 사용 예시 (0) | 2022.11.08 |
자바 이클립스 설정: 탭(Tab)을 공백(space) 4개로 변경하기 (0) | 2022.10.11 |
자바 해당 경로에 있는 모든 파일 압축하는 방법 (0) | 2022.10.11 |
자바 StringTokenizer 클래스 정의 및 사용 방법 (split 차이) (0) | 2022.09.20 |